File Receiver Python Script
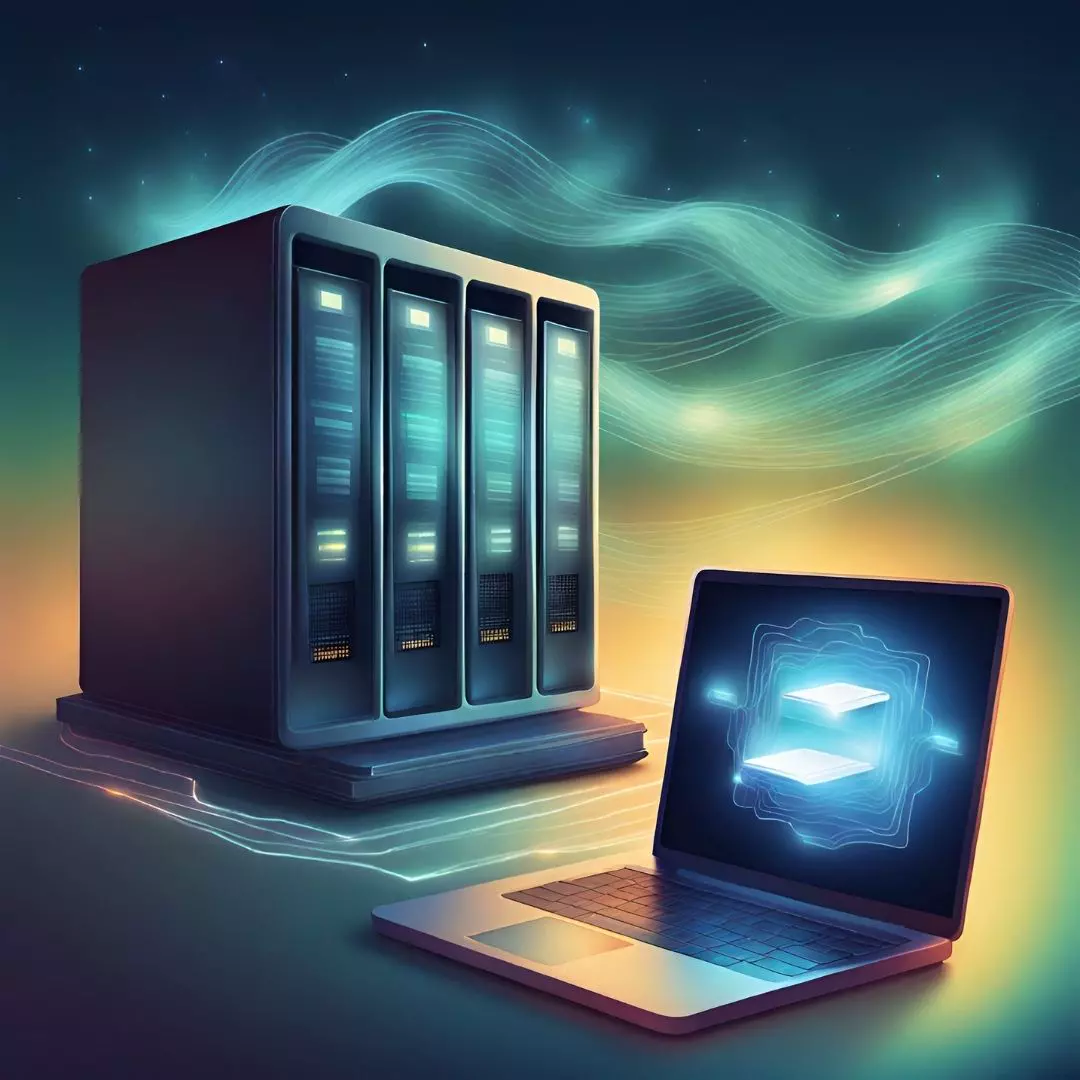
Overview
In this blog, you will see how to create a Python script that accepts files and how to send files via the curl
command from macOS and Linux computers.
Sometimes you may find yourself in cases where you are only able to connect to a server one way because of the access rules of the network.
For example, you have a server in the cloud and a laptop on your home network. And you want to send files from your laptop to the server. You can't start an HTTP server on the laptop and get the files from the server. You should start a file receiver
tool on the server and connect from your laptop to the server.
Pre-Requirements
🚫 You should know that this is not a production-ready solution for file uploading.
If you have SSH access, you can easily copy files with the scp
command from your local computer to the remote computer.
This Python script is only for edge cases.
- You should have
curl
installed on your computer that will upload the files. - Python3 should be installed on the remote computer that will receive the files.
- There should be at least one TCP port that is allowed between two computers. For example, TCP 80 port.
File Receiver Python Script on Remote Computer
First, you should SSH into the computer you want to upload. Then you can start the python script and wait for files to be uploaded. You can follow the steps below.
SSH into the remote computer
-i "$HOME/.ssh/aws"
: Private key that I use for connecting to the server.ubuntu
: Username on the computer.3.76.12.210
: Ip address of the computer.
ssh -i "$HOME/.ssh/aws" [email protected]
Create a file named file-receiver.py
with vim
.
vim file-receiver.py
Copy this python script and paste into the file-receiver.py
file.
import http.server
import socketserver
import os
# Set the port number you want to use
PORT = 80
# Set the chunk size (in bytes)
CHUNK_SIZE = 1024 * 1024 # 1MB
# Create a custom request handler to handle file uploads
class CustomRequestHandler(http.server.BaseHTTPRequestHandler):
def do_POST(self):
try:
# Get the total content length
content_length = int(self.headers['Content-Length'])
# Get the file name from the URL path
file_name = os.path.basename(self.path)
# Open the file for writing in binary mode
with open(file_name, 'wb') as file:
remaining_size = content_length
# Read and write the file in chunks
while remaining_size > 0:
chunk_size = min(CHUNK_SIZE, remaining_size)
chunk = self.rfile.read(chunk_size)
file.write(chunk)
remaining_size -= len(chunk)
self.send_response(200)
self.end_headers()
self.wfile.write(b'File uploaded successfully')
except Exception as e:
self.send_response(500)
self.end_headers()
self.wfile.write(f'Error: {str(e)}'.encode())
# Create the HTTP server with the custom request handler
with socketserver.TCPServer(("", PORT), CustomRequestHandler) as httpd:
print(f"Serving on port {PORT}")
httpd.serve_forever()
Run the server with sudo.
- If you have used a port number lower than 1024, then you must run the script with sudo.
- Otherwise you don't need sudo to run the script.
- If you get
Address already in use
, that means another application is already running on the port you choose. Kill the other app or choose another port.
sudo python3 file-receiver.py
Once you see an output like below, that means remote computer is ready to accept files.
$ sudo python3 file-receiver.py
Serving on port 80
Uploading Files From Local Computer
Once your file receiver
script is running on the remote server, you can start uploading files from local computer.
Open a terminal and change directory to same with the files you want to upload.
cd ~/Downloads
Now you can upload any file with the curl
command.
--upload-file sonarcube.pdf
: File to be uploaded.http://3.76.7.240:80
: IP and port of the remote computer./sonarcube.pdf
: The file name that uploaded file will be saved as.
curl -X POST --upload-file sonarcube.pdf http://3.76.7.240:80/sonarcube.pdf
When you run the curl
command, you will see an output message.
File uploaded successfully
Checking Files on the Remote Computer
After you run the curl command and see the File uploaded successfully
output, you can check the remote computer about what's going on.
If you check the python script logs, you will a line about the file upload.
78.190.2.120 - - [24/Feb/2024 10:22:38] "POST /sonarcube.pdf HTTP/1.1" 200 -
Like you see in the output above, that means your remote computer received the files successfully.
You can press ctrl+c
to stop the python server.
To see the received files, you can list the current directory.
ls -l
If you see the file, congratulations!